Spring Boot Login Page
last modified July 31, 2023
In this article we show how to work with a default login page. Spring security secures all HTTP endpoints by default. A user has to login in a default HTTP form.
To enable Spring Boot security, we add spring-boot-starter-security
to the dependencies.
Spring Boot Login Page example
The following example shows how to set up a simple login page in a Spring Boot application.
build.gradle ... src ├───main │ ├───java │ │ └───com │ │ └───zetcode │ │ │ Application.java │ │ └───controller │ │ MyController.java │ └───resources │ application.properties └───test └───java
This is the project structure of the Spring Boot application.
plugins { id 'org.springframework.boot' version '3.1.1' id 'io.spring.dependency-management' version '1.1.0' id 'java' } group = 'com.zetcode' version = '0.0.1-SNAPSHOT' sourceCompatibility = '17' repositories { mavenCentral() } dependencies { implementation 'org.springframework.boot:spring-boot-starter-web' implementation 'org.springframework.boot:spring-boot-starter-security' }
This is the Gradle build file. We have starters for web and security.
spring.main.banner-mode=off logging.pattern.console=%d{dd-MM-yyyy HH:mm:ss} %magenta([%thread]) %highlight(%-5level) %logger.%M - %msg%n
In the application.properties
file, we turn off the Spring Boot
banner and configure the console logging pattern.
package com.zetcode.controller; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @RestController public class MyController { @GetMapping("/") public String home() { return "This is home page"; } }
We have a simple home page.
We run the application and navigate to localhost:8080
. We are
redirected to the http://localhost:8080/login
page.
... Using generated security password: df7ce50b-abae-43a1-abe1-0e17fd81a454 ...
In the console, we can see a password generated for a default user called
user
. These credentials are provided to the authentication form.
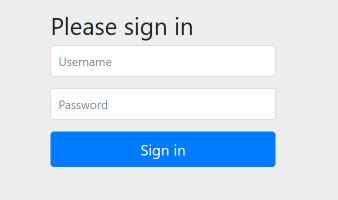
Spring uses Bootstrap to define the UI.
spring.security.user.name = admin spring.security.user.password = s$cret
With these two options, we can have a new user name and password. The autogenerated user is turned off with these settings.
In this article we have worked with a default login form.