Advanced Java Swing e-book
The e-book has 621 pages and 206 code examples. The e-book goes quickly to the point and is loaded with practical examples which focus on one important aspect. Heavy emphasis is placed on clarity and conciseness. No time is wasted with unimportant digressions. It is an ideal material for those who already know some basics of Java Swing. The e-book is written in plain English.
The code examples were tested on Linux and Windows. They can be run on JDK 7 and JDK 8. After purchasing the e-book, you will obtain a ZIP file. In the ZIP file, you will find a PDF file and all code examples. The code examples are in the form of NetBeans projects.
Once you make a payment, you will also get a confirmation email from Paypal. If something goes wrong with the payment process, drop me an email at vronskij(at)gmail.com.
Price
The e-book costs 21 €.
About this e-book
This is Advanced Java Swing e-book. This e-book covers advanced parts of the Swing in a great detail. The complete table of contents is listed at the end of the page.
This e-book is divided into three parts, which contain 12 chapters:
- Foundations
- Toplevel containers
- Events
- SwingWorker
- Painting
- BufferedImage
- Miscellaneous
- Layout management
- GroupLayout
- MigLayout
- Advanced components
- JList
- JTable
- JTree
- Text components
The Foundations part explains six different areas of Swing. The
first chapter of the e-book covers Swing toplevel
containers—fundamental components which contain other
Swing components. The Events chapter
is an in-depth coverage of the event handling system of Swing. It
has 25 examples. The SwingWorker chapter explains the very important
SwingWorker
class and provides three detailed examples.
The Painting chapter explains the basics of the painting
mechanism. In the BufferedImage chapter, we show how to work with images
in Swing. In the Miscellaneous chapter, we cover various aspects of Swing,
including keyboard handling, compound border, and making
a screenshot.
The Layout management part explains the layout management of
Swing components. This area is considered to be one of the most
difficult areas of GUI programming. Therefore, the e-book gives
particular emphasis on the layout management process. There are two
chapters: GroupLayout manager and MigLayout manager. These are the most
flexible layout managers available for Swing. The chapters contain many
easy to understand practical examples. (GroupLayout
18 examples,
MigLayout
41 examples.)
The Advanced components part covers advanced Swing components
in a great detail. Plenty of practical examples explain the
JList
(14 examples), the JTree
(22 examples),
the JTable
(29 examples), and several text
components (28 examples).
Screenshots
Here you can see some of the screenshots from the e-book.
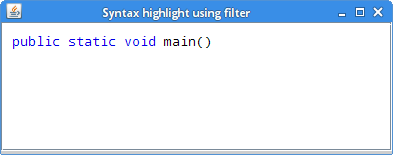
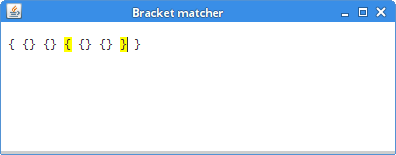
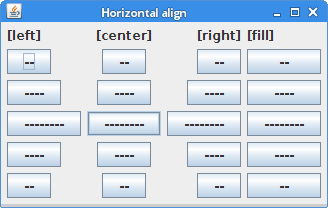
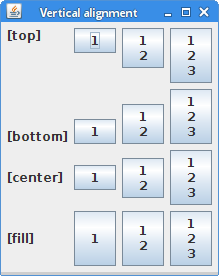
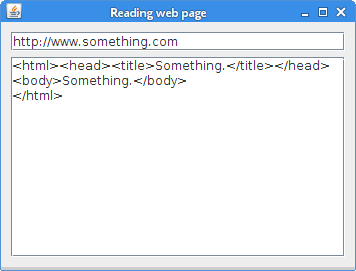
ExecutorService
.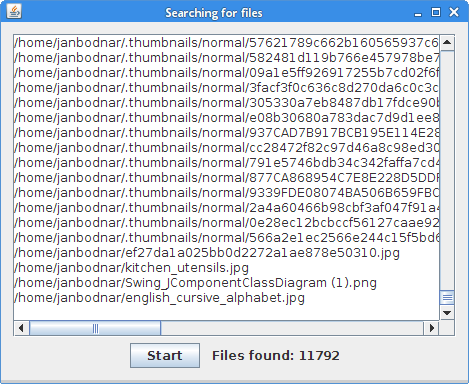
SwingWorker
to search for files.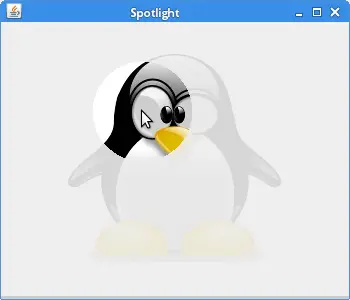
BufferedImage
to create a nice spotlight effect.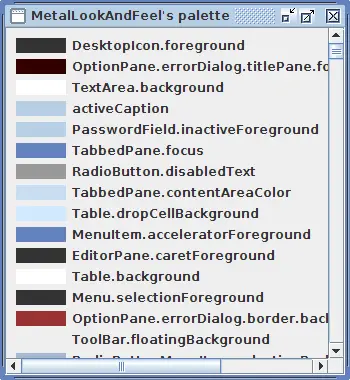
UIManager
.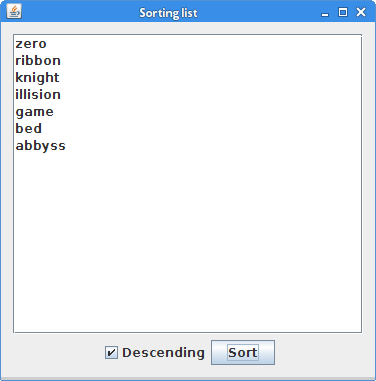
JList
component.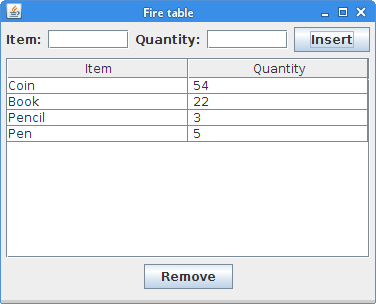
AbstractTableModel
.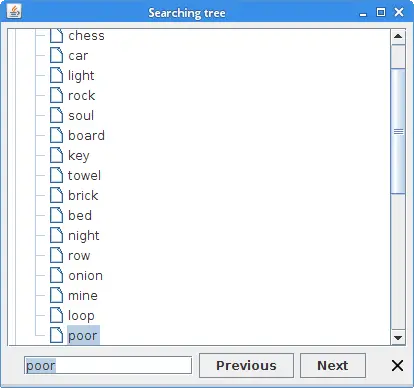
JTree
component.Table of Contents
- Preface
- About the author
- Foundations
- Toplevel containers
- JFrame
- JLayeredPane
- Glass pane
- JDialog
- JWindow
- Events
- Multiple listeners
- Multiple sources
- EventHandler
- Custom event
- Observer pattern
- Actions
- Action example
- Toggle buttons
- ActionListener vs ItemListener
- Application closing
- EventQueue
- AWTEventListener
- MouseEvent
- MouseListener
- Mouse event types
- MouseAdapter
- ItemEvent
- PaintEvent
- FocusEvent
- Selecting all text on gaining focus
- Initial focus
- Scheduling tasks
- Swing timer
- Utility timer
- Executor service
- SwingWorker
- SplashScreen
- Searching images
- Supervisor worker
- Painting
- The paintComponent() method
- Custom icon
- Painting into image
- Grayscale image
- Component capture
- The repaint() method
- Points
- Moving circle
- BufferedImage
- Grayscale image
- Scaling an image
- Spotlight
- Sun and cloud
- Subimage
- Miscellaneous
- Keyboard
- KeyListener
- Key bindings
- Mnemonics and accelerators
- UIManager
- Selecting laf
- Colour palette
- Compound border
- Custom border
- Screen capture
- Opaque
- Difference in meaning
- Three labels
- Keyboard
- Toplevel containers
- Layout management
- GroupLayout manager
- First examples
- Simple example
- Simple example 2
- Independent dimensions
- Grid of buttons
- Grid of buttons 2
- Gaps
- Fixed gaps
- Automatic gaps
- Resizable gaps
- Indent
- Alignments
- Horizontal alignment
- Vertical alignment
- Baseline alignment
- Parallel group's size
- Practical examples
- Corner buttons
- Password
- Windows
- Review
- Go To Class
- Tags
- First examples
- MigLayout manager
- Grid
- Cells
- Horizontal flow mode
- Vertical flow mode
- Flow mode in a cell
- Orientation
- No grid
- Bound size
- Component size
- Component size 2
- Gap size
- Constraints
- Units
- Insets
- Absolute positioning
- Relative positioning
- Placing components
- Fill
- Push
- Gaps
- Column gaps
- Size groups
- Dock
- Vertical alignment
- Horizontal alignment
- Indent
- Pad
- Span
- Skip
- Split
- Shrink
- Grow
- Hidemode
- Debug
- Practical examples
- Simple example
- Corner buttons
- Windows
- Windows 2
- New folder
- New folder 2
- New folder 3
- External tools
- Replace text
- Monopoly
- Grid
- GroupLayout manager
- Advanced components
- JList component
- Simple examples
- List orientation
- DefaultListModel
- Read-only data
- Modifying data
- AbstractListModel
- Read-only data
- Modifying data
- ListDataListener
- Selections
- Renderers
- Rendering stripped background
- Rendering icons
- Rendering check boxes
- Sorting
- Filtering
- JTable component
- Simple table
- Grid lines
- DefaultTableModel
- Simple example
- Read-only table
- AbstractTableModel
- Simple example
- Firing data changes
- TableModelListener
- Selection model
- Table cell renderers
- Cell alignments
- The getColumnClass() method
- The prepareRenderer() method
- Custom cell renderer
- Number cell renderer
- Drawing icons
- Table cell editors
- ComboBoxEditor
- Custom cell age editor
- Currency editor
- Table columns
- Multi-line column headers
- TableColumnModelListener
- Adding and removing columns
- Column highlighting
- Table header renderer
- Sorting
- Automatic sorting
- Sorting months
- Sorting order
- Filtering
- Numeric filter
- Converting row indexes
- Row headers
- Final example
- JTree component
- Simple tree
- DefaultMutableTreeNode
- Tree creation
- Sorted tree
- DefaultTreeModel
- TreePath
- Tree rows
- Selections
- TreeSelectionListener
- Programmatically selecting nodes
- Adding and removing selections
- User object
- Tree enumerations
- Expanding and collapsing nodes
- Programmatically expanding and collapsing nodes
- TreeExpansionListener
- Tree cell renderers
- Tree cell editors
- DefaultCellEditor
- DefaultTreeCellEditor
- Editing phases
- Click, pause, click, delay
- Programmatically editing nodes
- Lazy loading
- Lazy loading with a TreeModel
- Lazy loading with a TreeWillExpandListener
- Searching tree
- Text components
- Appending text
- JPasswordField
- Text selection
- Line & column numbers
- Verifying input
- JFormattedTextField
- Inputverifier
- DocumentListener
- DocumentEvent
- PlainDocument
- Inserting text
- Character limit
- Element walker
- EditorKit
- View
- Simple view
- Coordinate system
- Word count
- Highlighting text
- Simple highlight
- Searching text
- Bracket matching
- NavigationFilter
- DocumentFilter
- Size filter
- Auto-indent
- Overwrite filter
- Text undo & redo
- Styles
- StyledEditorKit
- Syntax highlighting
- JList component
- Bibliography