PyQt QShortcut
last modified August 24, 2023
In this article we show how to work with QShortcut.
Sources are available at PyQt-Examples repository. Visit Advanced PyQt5 e-book, read PyQt5 tutorial, or list all PyQt tutorials.
A keyboard shortcut is a series of one or several keys that performs an action in the application.
QShortcut
QShortcut
is used to connect keyboard shortcuts to PyQt signals and
slots mechanism, so that objects can be informed when a shortcut is executed.
When the user types the key sequence for a given shortcut, the shortcut's
activated
signal is emitted.
The shortcut can be set up to contain all the key presses necessary to describe a keyboard shortcut, including the states of modifier keys such as Shift, Ctrl, Alt.
On certain widgets, using &
in front of a character automatically
creates a mnemonic (a shortcut is sometimes called mnemonic in this context)
for that character. For instance, &Quit
creates the shortcut Alt + Q. On Linux, the key is underlined.
On Windows, the key is underlined after we press the Alt key.
On Mac, mnemonics are disabled by default.
On widgets that do not support automatic mnemonics, we create a shortcut with
QShortcut
and QKeySequence
.
PyQt QShortcut simple example
The following example creates two shortcuts.
#!/usr/bin/python from PyQt5.QtWidgets import QWidget, QShortcut, QApplication, QMessageBox from PyQt5.QtGui import QKeySequence import sys class Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): self.msgSc = QShortcut(QKeySequence('Ctrl+M'), self) self.msgSc.activated.connect(lambda : QMessageBox.information(self, 'Message', 'Ctrl + M initiated')) self.quitSc = QShortcut(QKeySequence('Ctrl+Q'), self) self.quitSc.activated.connect(QApplication.instance().quit) self.setGeometry(300, 300, 300, 200) self.setWindowTitle('Shortcuts') self.show() def main(): app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_()) if __name__ == '__main__': main()
The Ctrl + M shortcut shows a message box and the Ctrl + Q quits the application.
self.msgSc = QShortcut(QKeySequence('Ctrl+M'), self)
The Ctrl + M shortcut is created. It is applied to the main window widget.
self.msgSc.activated.connect(lambda : QMessageBox.information(self, 'Message', 'Ctrl + M initiated'))
We connect a lambda function displaying a message box to the activated
signal of the shortcut.
self.quitSc = QShortcut(QKeySequence('Ctrl+Q'), self) self.quitSc.activated.connect(QApplication.instance().quit)
The Ctrl + Q shortcut terminates the application.
QShortcut on QPushButton
In the following example, we create a mnemonic shortcut on a push button.
#!/usr/bin/python from PyQt5.QtWidgets import (QWidget, QHBoxLayout, QApplication, QPushButton, QMessageBox, QSizePolicy) from PyQt5.QtGui import QKeySequence from PyQt5.QtCore import Qt import sys class Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): hbox = QHBoxLayout() msgBtn = QPushButton('&Show message', self) msgBtn.clicked.connect(lambda : QMessageBox.information(self, 'Message', 'Information message')) hbox.addWidget(msgBtn) msgBtn.setSizePolicy(QSizePolicy.Fixed, QSizePolicy.Fixed) hbox.setAlignment(Qt.AlignLeft) self.setLayout(hbox) self.move(300, 300) self.setWindowTitle('Shortcuts') self.show() def main(): app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_()) if __name__ == '__main__': main()
A message box is displayed when pressing the Alt + S shortcut.
msgBtn = QPushButton('&Show message', self)
The Alt + S shortcut is created by using the &
character in the label of the button.
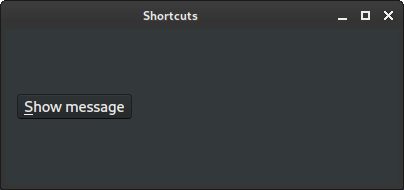
QShortcut with QAction and QMenu
We can create shortcuts with QAction
and QMenu
.
#!/usr/bin/python import sys from PyQt5.QtWidgets import QMainWindow, QAction, qApp, QApplication from PyQt5.QtGui import QIcon class Example(QMainWindow): def __init__(self): super().__init__() self.initUI() def initUI(self): exitAct = QAction(QIcon('exit.png'), '&Exit', self) exitAct.setShortcut('Ctrl+Q') exitAct.setStatusTip('Exit application') exitAct.triggered.connect(qApp.quit) self.statusBar() menubar = self.menuBar() fileMenu = menubar.addMenu('&File') fileMenu.addAction(exitAct) self.setGeometry(300, 300, 300, 200) self.setWindowTitle('Simple menu') self.show() def main(): app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_()) if __name__ == '__main__': main()
The example has three shortcuts: Alt + F for opening the File menu and then Alt + E for exiting the application. These shortcuts must be activated in the specified sequence. The Ctrl + Q terminates the application without needing to work with the menu.
exitAct = QAction(QIcon('exit.png'), '&Exit', self)
The Alt + E mnemonic is created by using the &
in the label of the QAction
.
exitAct.setShortcut('Ctrl+Q')
A global shortcut is created with the setShortcut
function.
fileMenu = menubar.addMenu('&File')
We create the Alt + F mnemonic with the addMenu
function.
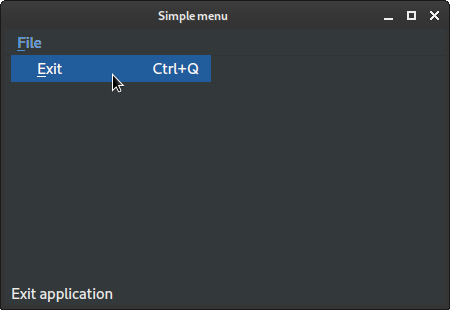
In this article we have presented the PyQt shortcuts.
Author
List all PyQt tutorials\.