Events and signals in Qt5
last modified October 18, 2023
In this part of the Qt5 C++ programming tutorial we talk about events and signals.
Events are an important part in any GUI program. All GUI applications are event-driven. An application reacts to different event types which are generated during its life. Events are generated mainly by the user of an application. But they can be generated by other means as well, e.g. Internet connection, window manager, or a timer. In the event model, there are three participants:
- event source
- event object
- event target
The event source is the object whose state changes. It generates Events. The event object (Event) encapsulates the state changes in the event source. The event target is the object that wants to be notified. Event source object delegates the task of handling an event to the event target.
When we call the application's exec
method, the application enters
the main loop. The main loop fetches events and sends them to the objects. Qt
has a unique signal and slot mechanism. This signal and slot mechanism is an
extension to the C++ programming language.
Signals and slots are used for communication between objects. A signal is emitted when a particular event occurs. A slot is a normal C++ method; it is called when a signal connected to it is emitted.
Qt5 click example
The first example shows a very simple event handling example. We have one push button. By clicking on the push button, we terminate the application.
#pragma once #include <QWidget> class Click : public QWidget { public: Click(QWidget *parent = nullptr); };
This is the header file.
#include <QPushButton> #include <QApplication> #include <QHBoxLayout> #include "click.h" Click::Click(QWidget *parent) : QWidget(parent) { auto *hbox = new QHBoxLayout(this); hbox->setSpacing(5); auto *quitBtn = new QPushButton("Quit", this); hbox->addWidget(quitBtn, 0, Qt::AlignLeft | Qt::AlignTop); connect(quitBtn, &QPushButton::clicked, qApp, &QApplication::quit); }
We display a QPushButton
on the window.
connect(quitBtn, &QPushButton::clicked, qApp, &QApplication::quit);
The connect
method connects a signal to the slot. When we click on
the Quit button, the clicked
signal is generated. The
qApp
is a global pointer to the application object. It is
defined in the <QApplication>
header file. The
quit
method is called when the clicked signal is emitted.
#include <QApplication> #include "click.h" int main(int argc, char *argv[]) { QApplication app(argc, argv); Click window; window.resize(250, 150); window.setWindowTitle("Click"); window.show(); return app.exec(); }
This is the main file.
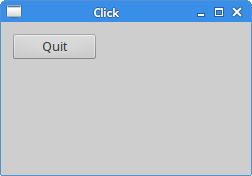
Qt5 KeyPress
In the following example, we react to a key press.
#pragma once #include <QWidget> class KeyPress : public QWidget { public: KeyPress(QWidget *parent = 0); protected: void keyPressEvent(QKeyEvent * e); };
This is the keypress.h
header file.
#include <QApplication> #include <QKeyEvent> #include "keypress.h" KeyPress::KeyPress(QWidget *parent) : QWidget(parent) { } void KeyPress::keyPressEvent(QKeyEvent *event) { if (event->key() == Qt::Key_Escape) { qApp->quit(); } }
The application terminates if we press the Escape key.
void KeyPress::keyPressEvent(QKeyEvent *e) { if (e->key() == Qt::Key_Escape) { qApp->quit(); } }
One of the ways of working with events in Qt5 is to reimplement an event handler.
The QKeyEvent
is an event object, which holds information about
what has happened. In our case, we use the event object to determine which key was
actually pressed.
#include <QApplication> #include "keypress.h" int main(int argc, char *argv[]) { QApplication app(argc, argv); KeyPress window; window.resize(250, 150); window.setWindowTitle("Key press"); window.show(); return app.exec(); }
This is the main file.
QMoveEvent
The QMoveEvent
class contains event parameters for move events.
Move events are sent to widgets that have been moved.
#pragma once #include <QMainWindow> class Move : public QWidget { Q_OBJECT public: Move(QWidget *parent = 0); protected: void moveEvent(QMoveEvent *e); };
This is the move.h
header file.
#include <QMoveEvent> #include "move.h" Move::Move(QWidget *parent) : QWidget(parent) { } void Move::moveEvent(QMoveEvent *e) { int x = e->pos().x(); int y = e->pos().y(); QString text = QString::number(x) + "," + QString::number(y); setWindowTitle(text); }
In our code programming example, we react to a move event. We determine the current x, y coordinates of the upper left corner of the client area of the window and set those values to the title of the window.
int x = e->pos().x(); int y = e->pos().y();
We use the QMoveEvent
object to determine the x
,
y
values.
QString text = QString::number(x) + "," + QString::number(y);
We convert the integer values to strings.
setWindowTitle(text);
The setWindowTitle
method sets the text to the title
of the window.
#include <QApplication> #include "move.h" int main(int argc, char *argv[]) { QApplication app(argc, argv); Move window; window.resize(250, 150); window.setWindowTitle("Move"); window.show(); return app.exec(); }
This is the main file.
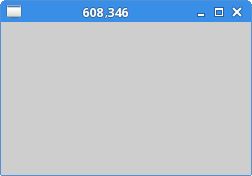
Disconnecting a signal
A signal can be disconnected from the slot. The next example shows how we can accomplish this.
#pragma once #include <QWidget> #include <QPushButton> class Disconnect : public QWidget { Q_OBJECT public: Disconnect(QWidget *parent = 0); private slots: void onClick(); void onCheck(int); private: QPushButton *clickBtn; };
In the header file, we have declared two slots. The slots
is not a C++ keyword, it is a Qt5 extension.
These extensions are handled by the preprocessor, before the code is compiled.
When we use signals and slots in our classes, we must provide
a Q_OBJECT
macro at the beginning of the
class definition. Otherwise, the preprocessor would complain.
#include <QTextStream> #include <QCheckBox> #include <QHBoxLayout> #include "disconnect.h" Disconnect::Disconnect(QWidget *parent) : QWidget(parent) { QHBoxLayout *hbox = new QHBoxLayout(this); hbox->setSpacing(5); clickBtn = new QPushButton("Click", this); hbox->addWidget(clickBtn, 0, Qt::AlignLeft | Qt::AlignTop); QCheckBox *cb = new QCheckBox("Connect", this); cb->setCheckState(Qt::Checked); hbox->addWidget(cb, 0, Qt::AlignLeft | Qt::AlignTop); connect(clickBtn, &QPushButton::clicked, this, &Disconnect::onClick); connect(cb, &QCheckBox::stateChanged, this, &Disconnect::onCheck); } void Disconnect::onClick() { QTextStream out(stdout); out << "Button clicked" << endl; } void Disconnect::onCheck(int state) { if (state == Qt::Checked) { connect(clickBtn, &QPushButton::clicked, this, &Disconnect::onClick); } else { disconnect(clickBtn, &QPushButton::clicked, this, &Disconnect::onClick); } }
In our example, we have a button and a check box. The check box connects and disconnects a slot from the buttons clicked signal. This example must be executed from the command line.
connect(clickBtn, &QPushButton::clicked, this, &Disconnect::onClick); connect(cb, &QCheckBox::stateChanged, this, &Disconnect::onCheck);
Here we connect signals to our user defined slots.
void Disconnect::onClick() { QTextStream out(stdout); out << "Button clicked" << endl; }
If we click on the Click button, we send the "Button clicked" text to the terminal window.
void Disconnect::onCheck(int state) { if (state == Qt::Checked) { connect(clickBtn, &QPushButton::clicked, this, &Disconnect::onClick); } else { disconnect(clickBtn, &QPushButton::clicked, this, &Disconnect::onClick); } }
Inside the onCheck
slot, we connect or disconnect the
onClick
slot from the Click button, depending on the received state.
#include <QApplication> #include "disconnect.h" int main(int argc, char *argv[]) { QApplication app(argc, argv); Disconnect window; window.resize(250, 150); window.setWindowTitle("Disconnect"); window.show(); return app.exec(); }
This is the main file.
Timer
A timer is used to implement single shot or repetitive tasks. A good example where we use a timer is a clock; each second we must update our label displaying the current time.
#pragma once #include <QWidget> #include <QLabel> class Timer : public QWidget { public: Timer(QWidget *parent = 0); protected: void timerEvent(QTimerEvent *e); private: QLabel *label; };
This is the header file.
#include "timer.h" #include <QHBoxLayout> #include <QTime> Timer::Timer(QWidget *parent) : QWidget(parent) { QHBoxLayout *hbox = new QHBoxLayout(this); hbox->setSpacing(5); label = new QLabel("", this); hbox->addWidget(label, 0, Qt::AlignLeft | Qt::AlignTop); QTime qtime = QTime::currentTime(); QString stime = qtime.toString(); label->setText(stime); startTimer(1000); } void Timer::timerEvent(QTimerEvent *e) { Q_UNUSED(e); QTime qtime = QTime::currentTime(); QString stime = qtime.toString(); label->setText(stime); }
In our example, we display a current local time on the window.
label = new QLabel("", this);
To display a time, we use a label widget.
QTime qtime = QTime::currentTime(); QString stime = qtime.toString(); label->setText(stime);
Here we determine the current local time. We set it to the label widget.
startTimer(1000);
We start the timer. Every 1000 ms a timer event is generated.
void Timer::timerEvent(QTimerEvent *e) { Q_UNUSED(e); QTime qtime = QTime::currentTime(); QString stime = qtime.toString(); label->setText(stime); }
To work with timer events, we must reimplement the timerEvent
method.
#include <QApplication> #include "timer.h" int main(int argc, char *argv[]) { QApplication app(argc, argv); Timer window; window.resize(250, 150); window.setWindowTitle("Timer"); window.show(); return app.exec(); }
This is the main file.
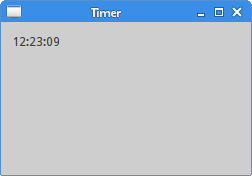
This chapter was dedicated to events and signals in Qt5.