Document.querySelector Tutorial
last modified Last modified: March 20, 2025
In this article, we demonstrate how to use querySelector
to select
HTML elements in JavaScript with practical examples.
Document.querySelector
The querySelector
method, part of the Document object, retrieves
the first element in the document matching a specified CSS selector. If no match
is found, it returns null
.
In contrast, querySelectorAll
returns a static
NodeList
containing all elements that match the given selector
group. This list does not update with document changes.
Document.querySelector Example
The following example illustrates how to use both
querySelector
and querySelectorAll
to manipulate HTML elements dynamically.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document.querySelector</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/semantic-ui/2.5.0/components/button.min.css"> <style> body { margin: 3em } .selected { background-color: #eee } .container { display: grid; grid-template-columns: 100px 100px 100px 100px 100px; grid-template-rows: 50px; grid-column-gap:5px; margin-bottom: 1em; } div>div { border: 1px solid #ccc; } </style> </head> <body> <div class="container"> <div></div> <div></div> <div></div> <div></div> <div></div> </div> <button id="first" type="submit" class="ui grey button">First</button> <button id="all" type="submit" class="ui brown button">All</button> <button id="clear" type="submit" class="ui brown button">Clear</button> <script src="main.js"></script> </body> </html>
This HTML document features five bordered div
elements within a container, styled as a grid.
Three buttons trigger visual changes to these div
s.
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/semantic-ui/2.5.0/components/button.min.css">
We enhance the document's appearance using the Semantic UI framework for consistent button styling.
.selected { background-color: #eee }
The .selected
class applies a light gray
background to highlighted div
elements.
.container { display: grid; grid-template-columns: 100px 100px 100px 100px 100px; grid-template-rows: 50px; grid-column-gap:5px; margin-bottom: 1em; }
The .container
class uses CSS Grid to
arrange five div
s in a single row with
equal column widths and a small gap between them.
div>div { border: 1px solid #ccc; }
Inner div
s within the container have a
light gray border for clear visual separation.
<div class="container"> <div></div> <div></div> <div></div> <div></div> <div></div> </div>
Five empty div
elements are nested in a
parent div
with the container
class.
<button id="first" type="submit" class="ui grey button">First</button> <button id="all" type="submit" class="ui brown button">All</button> <button id="clear" type="submit" class="ui brown button">Clear</button>
Three buttons control the div
elements:
"First" highlights the first div
, "All"
highlights all div
s, and "Clear" removes
the highlights. Semantic UI styles enhance their look.
<script src="main.js"></script>
The JavaScript logic resides in an external
main.js
file, linked at the document's end.
document.getElementById("first").onclick = (e) => { let tag = document.querySelector(".container div:first-child"); tag.className = "selected"; }; document.getElementById("all").onclick = (e) => { let tags = document.querySelectorAll(".container div"); tags.forEach( tag => { tag.className = "selected"; }); }; document.getElementById("clear").onclick = (e) => { let tags = document.querySelectorAll(".container div"); tags.forEach( tag => { tag.classList.remove("selected"); }); };
The main.js
file defines the behavior for each button, using
querySelector
and querySelectorAll
to manipulate the
DOM.
document.getElementById("first").onclick = (e) => {
An event listener is attached to the "First" button
using the onclick
property, selected via
getElementById
.
let tag = document.querySelector(".container div:first-child");
The querySelector
method targets the
first div
inside the .container
using the :first-child
pseudo-class.
tag.className = "selected";
The selected
class is assigned to the
targeted div
, applying the gray background.
let tags = document.querySelectorAll(".container div");
The querySelectorAll
method retrieves
all div
elements within the .container
as a NodeList
.
tags.forEach( tag => { tag.className = "selected"; });
Using forEach
, we iterate over the
NodeList
and apply the selected
class to each div
element.
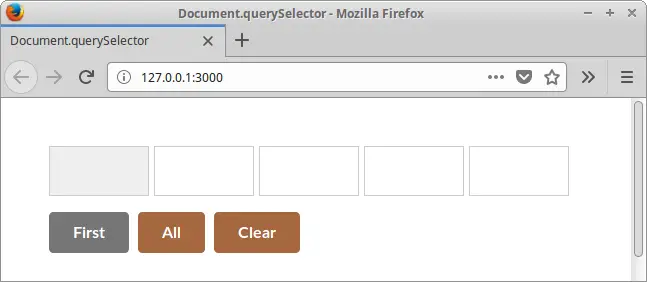
This screenshot shows the first div
highlighted with a gray background after clicking
the "First" button, demonstrating the effect.
Source
Document: querySelector Method
In this tutorial, we explored the use of
querySelector
and querySelectorAll
for selecting and manipulating HTML elements.