Node Sass tutorial
last modified last modified October 18, 2023
In this article we show how to work with a node-sass module. The node-sass module is used to translate the Sass code into CSS code.
Sass
Sass is a preprocessor scripting language that is interpreted or compiled into Cascading Style Sheets (CSS). Sass contains two syntaxes. The older syntax uses indentation to separate code blocks and newline characters to separate rules. The newer syntax, SCSS, uses block formatting like CSS. It uses braces to denote code blocks and semicolons to separate lines within a block.
The indented syntax and SCSS files are traditionally given the extensions
.sass
and .scss
, respectively.
Node-sass
Node-sass is a library that provides binding for Node.js to LibSass, the C version of the popular stylesheet preprocessor, Sass. It allows us to natively compile SCSS files to CSS.
Node Sass example
In the following example, we create a simple web project that uses the
node-sass
module.
$ mkdir sass $ mkdir -p public/css
In the project directory, we create three subdirectories. In the
sass
directory, we have SCSS code. The SCSS code is translated
into CSS and moved into the public/css
directory.
$ npm init -y
We initiate a new Node application.
$ npm i node-sass
We install the node-sass
module. We use the module to watch the
SCSS files and automatically translate them into CSS code.
$ npm install -g live-server
In addition, we install live-server
, which is a little development
server with live reload capability.
{ "name": "nodesass-ex", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "sass": "node-sass -w sass -o public/css" }, "keywords": [], "author": "Jan Bodnar", "license": "BSD", "dependencies": { "node-sass": "^5.0.0" } }
In the package.json
file, we create a script that runs the
node-sass
module. It will watch the sass
directory and output the compiled code into the public/css
directory.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="css/main.css"> <title>Home page</title> </head> <body> <div class="container"> <h1>Bugs</h1> <table> <tr> <th>Bug name</th> <th>Description</th> </tr> <tr> <td>Assasin bug</td> <td>The assassin bug uses its short three-segmented beak to pierce its prey and eat it.</td> </tr> <tr> <td>Bed bug</td> <td>Bed bugs are parasitic insects in the that feed exclusively on blood.</td> </tr> <tr> <td>Carpet beetle</td> <td>Considered a pest of domestic houses and, particularly, natural history museums where the larvae may damage natural fibers and can damage carpets, furniture, clothing, and insect collections.</td> </tr> <tr> <td>Earwig</td> <td>Earwigs are mostly nocturnal and often hide in small, moist crevices during the day, and are active at night, feeding on a wide variety of insects and plants.</td> </tr> </table> </div> </body> </html>
This is an HTML file with some data. This document is styled with a CSS file.
<link rel="stylesheet" href="css/main.css">
The CSS code is loaded from css/main
directory.
$myfont: Georgia 1.1em; $table_head_col: #ccc; $table_row_col: #eee; $table_bor_col: #eee; $container_width: 700px; $first_col_width: 150px; div.container { margin: auto; font: $myfont; width: $container_width; } table { tr:nth-child(odd) {background: $table_row_col} td:first-child {width: $first_col_width} th { background-color: $table_head_col; } border: 1px solid $table_bor_col; }
This is our SCSS code. We style the container and the table. The code uses two important SCSS capabilities: variables and nesting.
The following commands are run in separate terminals; they start two running processes.
$ npm run sass
We run the sass
script.
$ live-server --open=public
Finally, we start the development server. Now modify the
sass/main.scss
file.
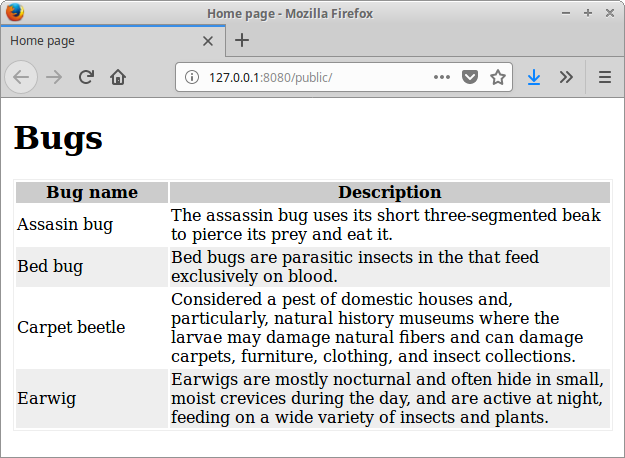
Source
In this article we have worked with the node-sass
module. We used the module in a simple web application to compile its SCSS
code into CSS code.