Transparency in HTML5 canvas
last modified July 17, 2023
In this part of the HTML5 canvas tutorial, we talk about transparency. We provide some basic definitions and two examples.
Transparency explained
Transparency is the quality of being able to see through a material. The easiest way to understand transparency is to imagine a piece of glass or water. Technically, the rays of light can go through the glass and this way we can see objects behind the glass.
In computer graphics, we can achieve transparency effects using alpha compositing. Alpha compositing is the process of combining an image with a background to create the appearance of partial transparency. The composition process uses an alpha channel. Alpha channel is an 8-bit layer in a graphics file format that is used for expressing translucency (transparency). The extra eight bits per pixel serves as a mask and represents 256 levels of translucency.
Transparent rectangles
The next example draws ten rectangles with different levels of transparency.
<!DOCTYPE html> <html> <head> <title>HTML5 canvas transparent rectangles</title> <script> function draw() { var canvas = document.getElementById('myCanvas'); var ctx = canvas.getContext('2d'); ctx.fillStyle = "blue"; for (var i = 1; i <= 10; i++) { var alpha = i * 0.1; ctx.globalAlpha = alpha; ctx.fillRect(50*i, 20, 40, 40); } } </script> </head> <body onload="draw();"> <canvas id="myCanvas" width="550" height="200"> </canvas> </body> </html>
We draw ten blue rectangles with various levels of transparency applied.
ctx.fillStyle = "blue";
The rectangles will be filled with blue colour.
var alpha = i * 0.1;
The alpha
value dynamically changes in the for loop.
In each loop, it is decreased by 10%.
ctx.globalAlpha = alpha;
The globalAlpha
property specifies the alpha value that
is applied to shapes and images before they are drawn onto the canvas.
The value is in the range from 0.0 (fully transparent) to 1.0 (fully opaque).
ctx.fillRect(50*i, 20, 40, 40);
The fillRect
method draws a filled rectangle. Its parameters are
x and y coordinates and width and height of the rectangle.
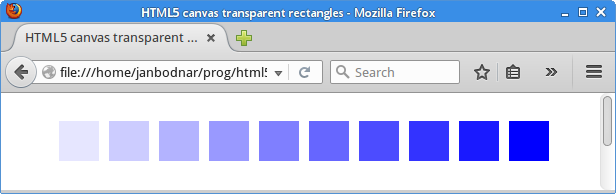
Fade out demo
In the next example we fade out an image. The image will gradually get more transparent until it is completely invisible.
<!DOCTYPE html> <html> <head> <title>HTML5 canvas fade out demo</title> <script> var alpha = 1.0; var ctx; var canvas; var img; function init() { canvas = document.getElementById('myCanvas'); ctx = canvas.getContext('2d'); img = new Image(); img.src = 'mushrooms.jpg'; img.onload = function() { ctx.drawImage(img, 10, 10); }; fadeOut(); } function fadeOut() { if (alpha <= 0) { return; } requestAnimationFrame(fadeOut); ctx.clearRect(0,0, canvas.width, canvas.height); ctx.globalAlpha = alpha; ctx.drawImage(img, 10, 10); alpha += -0.01; } </script> </head> <body onload="init();"> <canvas id="myCanvas" width="350" height="250"> </canvas> </body> </html>
The example is animated. In each animation cycle, the alpha value decreases and the image is redrawn.
img = new Image(); img.src = 'mushrooms.jpg'; img.onload = function() { ctx.drawImage(img, 10, 10); }
These lines load and display an image on the canvas.
fadeOut();
Inside the init
function, we call the fadeOut
function, which starts the animation.
if (alpha <= 0) { return; }
When the alpha
value reaches zero, the animation is terminated.
The fadeOut
function is not called anymore by the
requestAnimationFrame
function.
requestAnimationFrame(fadeOut);
The requestAnimationFrame
is a convenient function to create
animations. It tells the browser to perform an animation. The parameter is a
function to be invoked before the repaint. The browser chooses an optimal
frame rate for the animation.
ctx.clearRect(0,0, canvas.width, canvas.height);
The clearRect
method erases the canvas.
ctx.globalAlpha = alpha; ctx.drawImage(img, 10, 10);
A new global alpha value is set and the image is redraw, taking the new alpha value into consideration.
alpha += -0.01;
Finally, the alpha
is decreased. In the next animation cycle
the image is drawn with a decreased alpha
value.
In this part of the HTML5 canvas tutorial, we have talked about transparency.