Using jQuery DatePicker in JSP and PHP
last modified July 13, 2020
In jQuery DatePicker tutorial, we show how to use the jQuery DatePicker component. The chosen date is displayed in a in Java JSP and PHP pages.
jQuery is a fast, small, and feature-rich JavaScript library. It makes HTML document traversal and manipulation, event handling, animation, and Ajax much simpler with an easy-to-use API that works across a multitude of browsers. jQuery UI is a set of user interface widgets, effects, interactions, and themes built on top of the jQuery Library.
DatePicker
is one of the UI widgets of the jQuery UI
library.
It is used for selecting a date value.
Java web application
In the following example, we use a DatePicker
component to select a date.
The selected date is sent and displayed in a JSP page.
<!DOCTYPE html> <html> <head> <title>jQuery DatePicker</title> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css"> <script src="https://code.jquery.com/jquery-1.12.4.js"></script> <script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script> <script> $(function () { $("#datepicker").datepicker(); }); </script> </head> <body> <form action="showDate.jsp"> <label for="datepicker">Enter date:</label> <input type="text" name="selDate" id="datepicker"> <input type="submit" value="Submit"> </form> </body> </html>
In the index.html
file, we use the DatePicker
component
in a form tag. The action
attribute points to the showDate.jsp
page.
<link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css"> <script src="https://code.jquery.com/jquery-1.12.4.js"></script> <script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
We include the JavaScript libraries and CSS styles for the DatePicker
component.
<script> $(function () { $("#datepicker").datepicker(); }); </script>
The DatePicker
component is created.
<input type="text" name="selDate" id="datepicker">
The DatePicker
is tied to this input tag.
<%@page contentType="text/html" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Showing date</title> </head> <body> <p> The chosen date is <%= request.getParameter("selDate") %> </p> </body> </html>
In the showDate.jsp
file, the selected date is printed.
PHP application
The next instructions show how to adapt the example for a PHP application.
<form action="showDate.php">
The form's action attribute points to the showDate.php
file.
<?php echo "The chosen date is: " . $_GET["selDate"]; ?>
The date is printed.
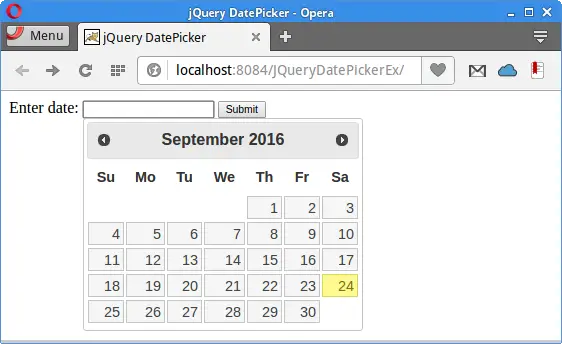
In this tutorial, we have used jQuery DatePicker in a Java web and PHP applications.